Execution of Java Program is the journey of turning your ideas into working applications. It starts with writing your code, moves through compiling it into bytecode, and ends with running it successfully.
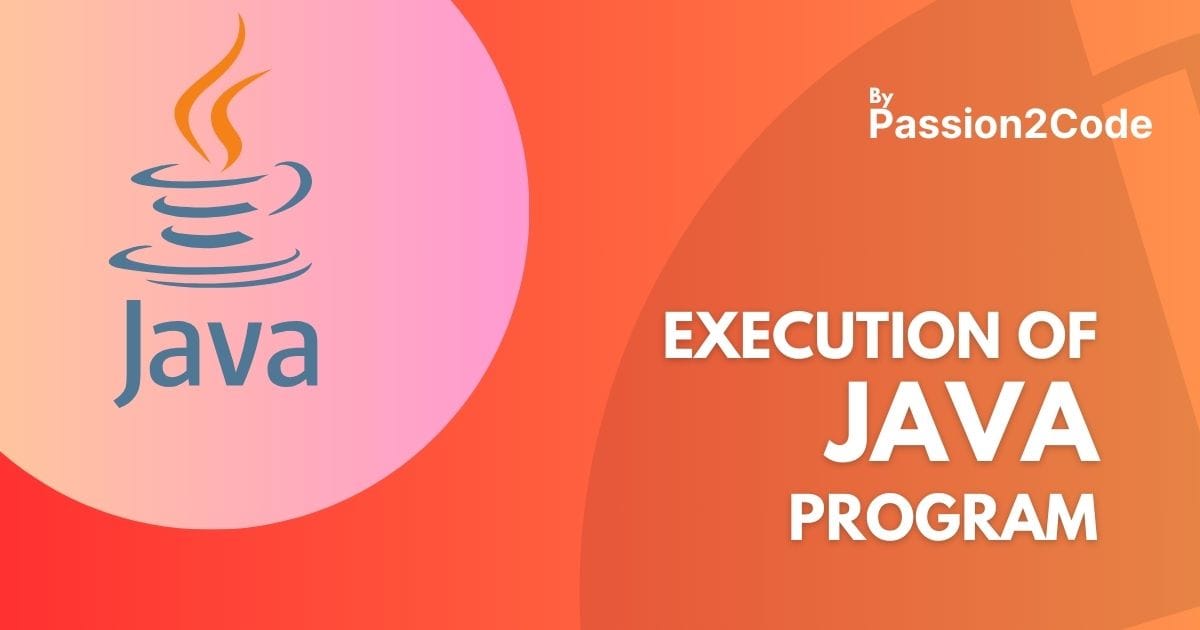
Execution of Java Program
Java is a powerful and high-level programming language known for its platform and robust performance. Understanding the Java process from writing code to executing it is fundamental for anyone who learning or working with Java.
- There are three main stages of the Java process: coding, compilation, and execution.
- Each step is crucial to converting your ideas into functional applications.
- Before understanding the Execution Process of a Java Program you must know what the Translator, Compiler, and Interpreter are and the types of files in Java.
Types of files in Java
There are two types of files in Java
- Java file
- Class file
Java file
- It is a file that stores the Java code and ends with the “.java” extension.
Class file
- It is a file that stores the byte code and ends with a “.class” extension.
What is Translator?
- It is a Machine/System that converts one language to another language.
What is a Compiler?

- A compiler is a translator that converts one language into another language at Once.
- This process is known as Compilation.
What is Interpreter?

- An interpreter is a translator that converts one language to another language Line by Line.
- This process is known as Interpretation/Execution.
Stages in the Execution of Java Program
There are three stages in the Execution of Java program. here,- Coding: Writing Java code
- Compilation
- Execution/Interpretation

Coding
- The process of writing a Java program/code to a Java file is known as coding.
- Writing code in Java, typically using a text editor or an integrated Development Environment (IDE).
- Java code is written in plain text files with extension .java.
- Syntax: Java is a statically typed language, so all variables and data types must be explicitly declared.
- Structure: Every Java program must include a .class file. The main() method serves as the entry point for execution.
- Tools for Coding:
- Popular IDEs: IntelliJ IDEA, Eclipse, and Visual Studio Code.
- Text Editors: Notepad++, Sublime Text, or even a basic text editor.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Compilation
- Once the code is written, the next step is compilation.
- After coding, the programmer needs to send the Java file to the compiler.
- The compiler will convert Java code into bytecode.
- Use the javac (Java compiler) tool provided in the JDK to compile the code.
- The compiler translates the .java file into a .class file containing bytecode.
- Before that conversion compiler will perform two operations.
- CTE – Compile Time Error, The computer will produce CTE only if the Java program is not correct as per Java rules.
- CTS – Compile Time Success, The Computer will produce CTS only if the Java program is correct as per Java rules.
- Command-Line Compilation: To compile a Java program, use the following command in the terminal or command prompt.
- javac Helloworld.java
- If there are no errors, the compiler produces CTS– CompileTime Success, .class file will be generated, which is Helloworld.class.
- Bytecode is platform-independent and can be executed on any system with a Java Virtual Machine(JVM).

Execution/Interpretation
- The final step is to execute the bytecode.
- It is the programmer’s responsibility to take the .class file and send it to the interpreter.
- This is done by using the Java Virtual Machine (JVM), which interprets the bytecode and translates it into machine-specific instructions.
- Use the java command to run the compiled .class file.
- The interpreter will take the .class file and it converts instructions into binary format.
- The JVM loads the bytecode, verifies it for security, and interprets or compiles it into native machine code (Just-In-time Compilation).
- The given instructions will be understandable by the machine according to the task that will be performed.
- Command-Line Execution: Run the following command:
- Java HelloWorld
- The output will be:
- Hello, world!
- The JVM ensures that the program runs in a secure and controlled environment.
- Execution involves multiple stages, including class loading, bytecode verification, and runtime interpretation.
Must Read
Expand your understanding of Java with these related articles:Structure of Java Program – Java Syntax Learn about the structure of a Java program and how to define variables using different data types effectively.JVM (Java Virtual Machine) – The Heart of Java Execution Explore how the JVM handles data types during program execution for efficient performance.JVM vs JRE vs JDK – What is the Difference Between JVM, JRE, and JDK? Understand how these components interact with Java data types to execute your programs.FAQ’s
What are the main stages of the process Execution of Java Program?
The Java process consists of three main stages:- Coding: Writing Java code in a .java file.
- Compilation: Using the Java compiler (javac) to convert the .java file into a .class file containing bytecode.
- Execution/Interpretation: Using the Java Virtual Machine (JVM) to run the bytecode and generate output.
What is the difference between a .java file and a .class file?
- A .java file contains human-readable Java code.
- A .class file contains bytecode, which is platform-independent and can be executed on any system with a JVM.
- CTE: Errors detected by the compiler when the code does not adhere to Java’s syntax and rules.
- CTS: Success indication when the code complies with Java’s syntax, allowing the compiler to generate a .class file.
- Integrated Development Environments (IDEs): IntelliJ IDEA, Eclipse, Visual Studio Code.
- Text Editors: Notepad++, Sublime Text, or a basic text editor.
- The JVM (Java Virtual Machine) is responsible for:
- Loading and verifying bytecode.
- Translating bytecode into machine code.
- Ensuring secure and optimized program execution.
- Why is Java considered platform-independent? Java achieves platform independence through its bytecode. The bytecode can run on any operating system or device with a compatible JVM, following the “Write Once, Run Anywhere” (WORA) principle.
- Syntax errors during coding, like missing semicolons or incorrect declarations.
- Compilation errors due to unrecognized code or incompatible types.
- Runtime errors during execution, such as null pointer exceptions or array index out-of-bounds errors.
- Syntax errors: Carefully review the error messages from the compiler and fix the code.
- Compilation errors: Ensure proper imports, variable declarations, and correct syntax.
- Runtime errors: Use debugging tools in your IDE or include print statements to track variable states and identify issues.